Public Class Form1
Dim lblDice1, lblDice2, lblDice3, lblDice4, lblDice5 As New Label
Dim WithEvents butRoll As New Button
Dim nYatzee, nFourOfAKind, nThreeOfAKind As New Integer
Dim lblYatzee, lblFourOfAKind, lblThreeOfAKind As New TextBox
Dim rnd As New Random
Private Sub addDice(ByRef lbl As Label, ByVal x As Integer, ByVal y As Integer)
lbl.Text = 0
lbl.Location = New Point(x, y)
lbl.Font = New Drawing.Font("Microsoft Sans Serif", 28.0F, System.Drawing.FontStyle.Regular, System.Drawing.GraphicsUnit.Point)
lbl.Height = 40
lbl.Width = 40
End Sub
Private Sub AddCount(ByRef txt As TextBox, ByVal x As Integer, ByVal y As Integer, ByVal z As String)
txt.Text = z
txt.Location = New Point(x, y)
txt.Width = 150
End Sub
Private Sub BC(ByVal x As Integer, ByVal y As Integer, ByVal z As String)
butRoll.Text = z
butRoll.Location = New Point(x, y)
End Sub
Private Sub Form1_Load(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles MyBase.Load
addDice(lblDice1, 10, 20)
addDice(lblDice2, 70, 20)
addDice(lblDice3, 130, 20)
addDice(lblDice4, 190, 20)
addDice(lblDice5, 250, 20)
AddCount(lblYatzee, 20, 140, "Yatzee: 0")
AddCount(lblFourOfAKind, 20, 180, "Four Of A Kind: 0")
AddCount(lblThreeOfAKind, 20, 220, "Three Of A Kind: 0")
BC(butRoll, 100, 90, "Roll")
Me.Controls.Add(lblDice1)
Me.Controls.Add(lblDice2)
Me.Controls.Add(lblDice3)
Me.Controls.Add(lblDice4)
Me.Controls.Add(lblDice5)
Me.Controls.Add(butRoll)
Me.Controls.Add(lblYatzee)
Me.Controls.Add(lblFourOfAKind)
Me.Controls.Add(lblThreeOfAKind)
End Sub
Private Sub RollDice() Handles butRoll.Click
Dim arrNumbers() As Integer = {0, 0, 0, 0, 0, 0}
lblDice1.Text = Rnd.Next(1, 7)
lblDice2.Text = Rnd.Next(1, 7)
lblDice3.Text = Rnd.Next(1, 7)
lblDice4.Text = Rnd.Next(1, 7)
lblDice5.Text = Rnd.Next(1, 7)
For Each lbl As Label In Me.Controls.OfType(Of Label)()
arrNumbers(lbl.Text - 1) += 1
Next
For Each i As Integer In arrNumbers
If i = 5 Then
nYatzee += 1
ElseIf i = 4 Then
nFourOfAKind += 1
ElseIf i = 3 Then
nThreeOfAKind += 1
End If
Next
lblYatzee.Text = "Yatzees: " & nYatzee
lblFourOfAKind.Text = "Four Of A Kind: " & nFourOfAKind
lblThreeOfAKind.Text = "Three Of A Kind: " & nThreeOfAKind
End Sub
Private Sub BC(ByVal button As Button, ByVal p2 As Integer, ByVal p3 As Integer, ByVal p4 As String)
Throw New NotImplementedException
End Sub
End Class
Fun
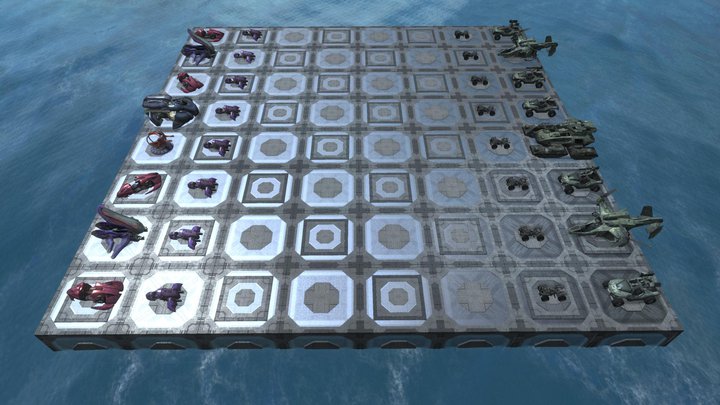
Want to Play!
Sunday, April 17, 2011
Yatzee!!!!!!
Labels:
vb.net
Subscribe to:
Post Comments (Atom)
No comments:
Post a Comment